I need use UICollectionView, but target my app for iOS < 6.0. So I’ve found PSTCollectionView library and using it with my rubymotion project. You can find whole source code in my github repo.
You need to download and link PSTCollectionView directory into vendor/PSTCollectionView .
Then update yourRakefile :
app.vendor_project('vendor/PSTCollectionView', :static)
app.frameworks += %w(QuartzCore UIKit)
app.deployment_target = "5.0"
app.device_family = [:ipad]
create app/controllers and add two files – cell.rb and view_controller.rb
cell.rb
class Cell < PSTCollectionViewCell #PSUICollectionViewCell
attr_accessor :label
def initWithFrame(frame)
if super
@label = UILabel.alloc.initWithFrame(CGRectMake(0.0, 0.0, frame.size.width, frame.size.height))
@label.autoresizingMask = UIViewAutoresizingFlexibleHeight | UIViewAutoresizingFlexibleWidth
@label.textAlignment = UITextAlignmentCenter
@label.font = UIFont.boldSystemFontOfSize(50.0)
@label.backgroundColor = UIColor.underPageBackgroundColor
@label.textColor = UIColor.whiteColor
self.contentView.addSubview(@label)
end
self
end
end
and view_controller.rb
class ViewController < PSTCollectionViewController #PSUICollectionViewController
def viewDidLoad
super
pinchRecognizer = UIPinchGestureRecognizer.alloc.initWithTarget(self, action:'handlePinchGesture')
self.collectionView.addGestureRecognizer(pinchRecognizer)
self.collectionView.registerClass(Cell, forCellWithReuseIdentifier: "MY_CELL")
end
def collectionView(view, numberOfItemsInSection: section)
63
end
def collectionView(collectionView, cellForItemAtIndexPath: indexPath)
cell = collectionView.dequeueReusableCellWithReuseIdentifier("MY_CELL", forIndexPath:indexPath)
cell.label.text = 'test'
cell
end
def collectionView(collectionView, layout: collectionViewLayout, sizeForItemAtIndexPath:indexPath)
CGSizeMake(120, 120)
end
def collectionView(collectionView, layout: collectionViewLayout, minimumInteritemSpacingForSectionAtIndex: section)
30
end
def collectionView(collectionView, layout:collectionViewLayout, minimumLineSpacingForSectionAtIndex: section)
30
end
end
Finally, change app_delegate.rb
class AppDelegate
def application(application, didFinishLaunchingWithOptions:launchOptions)
@window = UIWindow.alloc.initWithFrame UIScreen.mainScreen.bounds
view_controller = ViewController.alloc.initWithCollectionViewLayout(PSTCollectionViewFlowLayout.new)
@window.rootViewController = view_controller
@window.makeKeyAndVisible
true
end
end
runrake and voila! :)
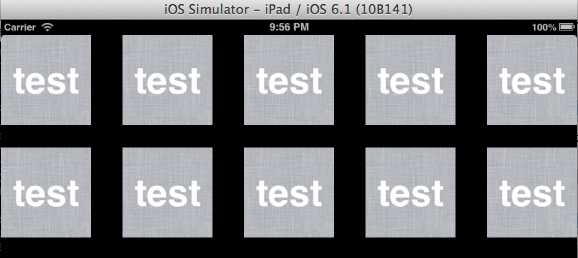